Community Plugin
View plugin on GitHub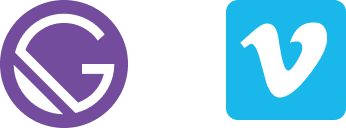
gatsby-source-vimeo-all
A way to get all of your vimeo videos using the vimeo node-sdk. Great if you don't want to embed the movies using iframe, but use them in a <video /> element.
Installation
npm i gatsby-source-vimeo-all
- Register an app on vimeo. You will need a clientId, a clientSecret and an accessToken. Access tokens can be generated on the vimeo app page.
- Add config to
gatsby-config.js
// gatsby-config.js
module.exports = {
plugins: [
//... Other plugins
{
resolve: 'gatsby-source-vimeo-all',
options: {
clientId: 'YOUR_CLIENT_ID',
clientSecret: 'YOUR_CLIENT_SECRET',
accessToken: 'YOUR_ACCESS_TOKEN'
}
}
]
}
Example usage
Bare bones example to get you started.
import React from 'react'
import { graphql, useStaticQuery } from 'gatsby'
const query = graphql`
{
vimeo(link: { eq: "https://vimeo.com/315401283/dfd80bf8c1" }) {
name
description
duration
link
aspectRatio
width
height
srcset {
...GatsbyVimeoSrcSet
}
pictures {
uri
}
user {
name
}
}
}
`
const Video = () => {
const { vimeo } = useStaticQuery(query)
return <video src={vimeo.srcset[0].link} controls autoPlay loop />
}
export default Video
Fragments
GatsbyVimeoSrcSet
Gives you all of the srcset properties. This is probably the one you’re going to use the most.